Using code smells to refactor to more OO code (an example with temporary field, solution sprawl and feature envy)
Published by Manuel Rivero on 14/03/2024
Introduction.
During our deliberate practice program[1], we were working on a modified version of the CoffeeMachine kata[2]. After some sessions we had implemented the following functionality documented by the tests:
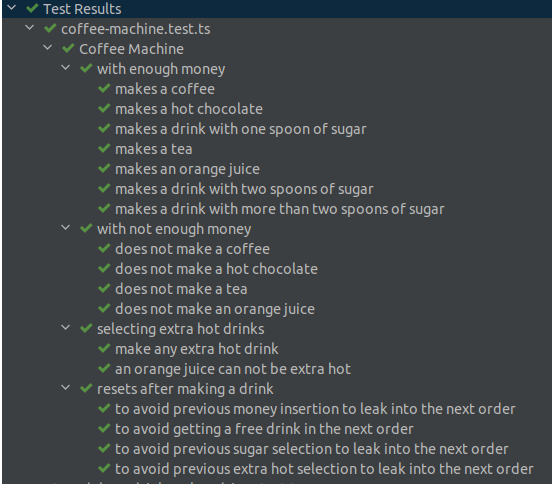
which was implemented by the following code in the CoffeeMachine
class: